Intro
In this article, I will show how to use ML.Net (Machine Learning framework for .Net developers) to create an API service that will analyze comments sentiments (e.g. positive or negative sentiment of the comment).
Prerequisites
You will need to install:
Create a simple API application
I am using Visual Studio 2019 so I will just create a new web api project through standard VS command. Visual Studio will generate a standard boilerplate for REST api application.
After boilerplate is ready right click on the project, select Add -> Machine Learning -> Text classification.
Data for training
We need some data to train our model. For training purposes we can use Microsoft’s training data for Wikipedia comments. You can download it here: Wikipedia detox dataset.
This file consists of 2 columns. The first one is sentiment score (1 – toxic or 0 – non-toxic) and the second is an actual comment left by user.
Import data in ML Builder Data Section. You should see something like this:
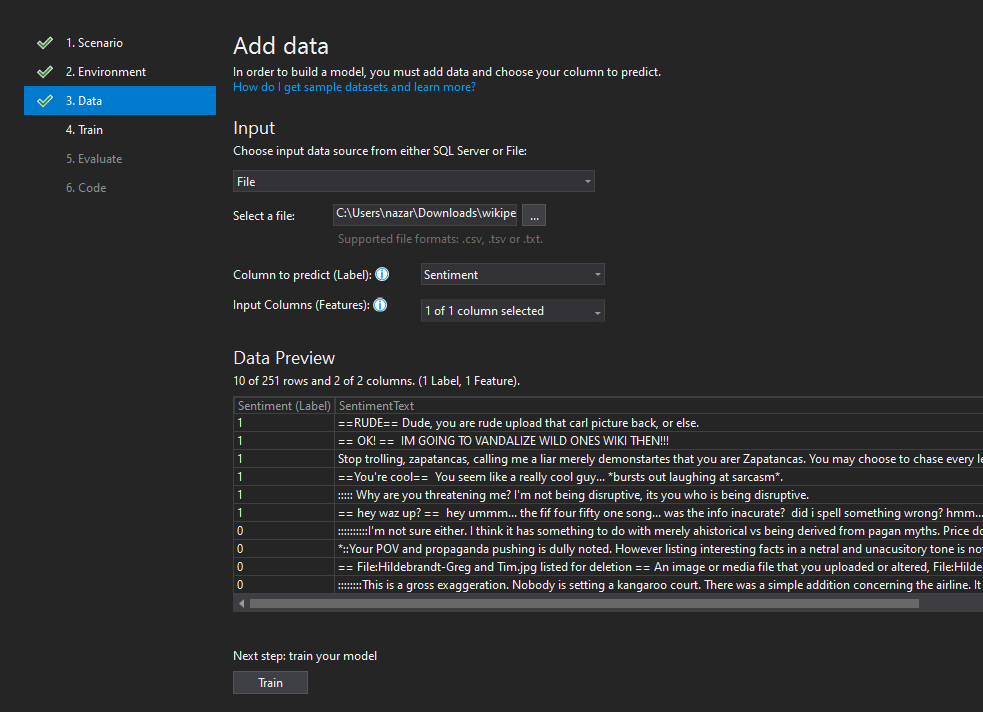
Train a model
You should understand how much time do you need to train your model. Of course, it depends on data complexity, scenarios, CPU performance, etc., but the general rule is the following:
Dataset size | Average time to train |
0 – 10 MB | 10 sec |
10 – 100 MB | 10 min |
100 – 500 MB | 30 min |
500 – 1 GB | 60 min |
1 GB+ | 3+ hours |
You can see more information in How long should I train for? guide.
Generate Code
Model Builder will generate code for using our model in separate project.
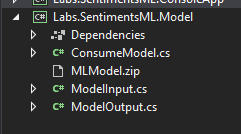
Let’s now use this code in our API.
Create Controller
Now we can create a controller to use a ML model. I will create a simple DTO class for returning object:
public class SentimentResultDto
{
public string SentimentValue { get; set; }
public string PredictionScore { get; set; }
}
The whole controller will look like this:
[Route("api/[controller]")]
[ApiController]
public class SentimentsController: ControllerBase
{
[HttpPost]
public IActionResult GetScore(SentimentTextDto sentimentDto)
{
var input = new ModelInput {SentimentText = sentimentDto.SentimentText};
var result = ConsumeModel.Predict(input);
var sentResultsDto = new SentimentResultDto
{
SentimentValue = result.Prediction,
PredictionScore = result.Score.First().ToString("n2")
};
return Ok(sentResultsDto);
}
}
Validate solution
Now we have an API service to check sentiments score. Let’s check it with the postman.
Toxic Comment
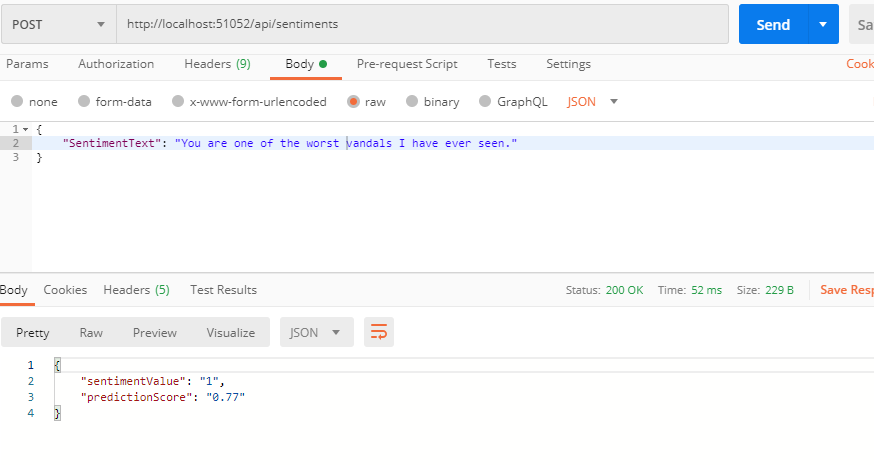
Non-Toxic Comment
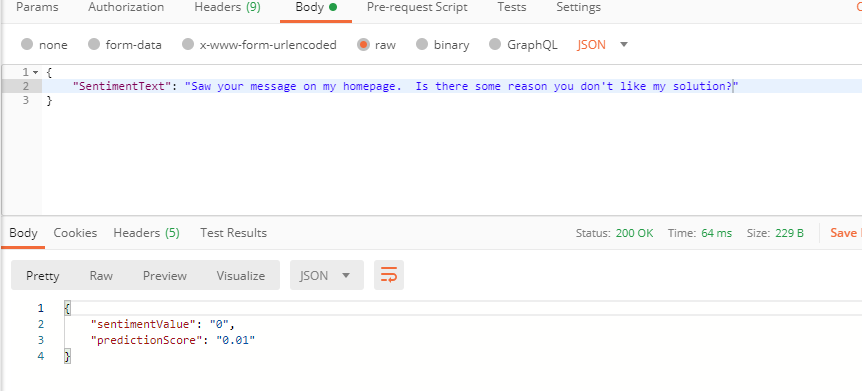