Today I want to share some guides on how to use SignalR library.
SignalR is an open-source library that simplifies adding real-time web functionality to apps. Real-time web functionality enables server-side code to push content to clients instantly without reloading the page. This can be useful if you want to create real-time Dashboards, notifications, email, chat, etc.
Backend
Create project
Let’s start with creating a backend. I will be using Visual Studio to create a simple .net core api application. Let’s call our project “Notify”.
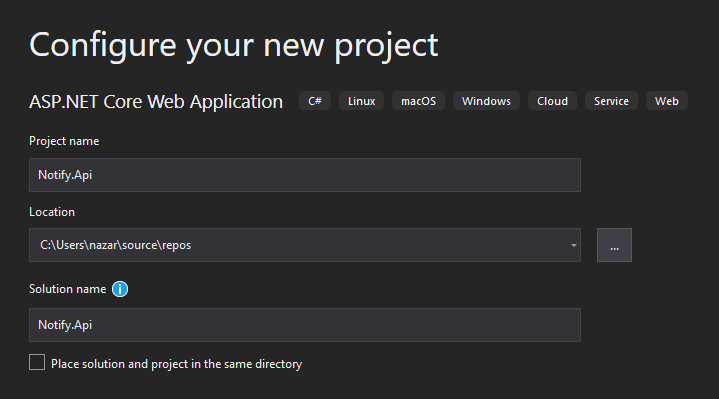
We will also need to install a SignalR library dependency. We can do it with the following command:
Install-Package Microsoft.AspNet.SignalR.Core -Version 2.4.1
Code the API
Firstly lets create simple model to represent our message:
namespace Notify.Api
{
public class NotifyMessage
{
public string Message { get; set; }
}
}
SignalR uses hubs to connect your api with a client web api. You just need to define a method that will be used by a client, SignalR will do the rest.
namespace Notify.Api
{
using System.Threading.Tasks;
using Microsoft.AspNetCore.SignalR;
public class Hubs : Hub
{
public async Task SendMessage(NotifyMessage message)
{
await Clients.All.SendAsync("ReceiveMessage", message);
}
}
}
This code will send a message to all clients that are listening to event “ReceiveMessage”.
The SignalR middleware requires some services, which are configured by calling services.AddSignalR
. Simply add this line to the ConfigurationService method in Startup.cs + create an endpoint:
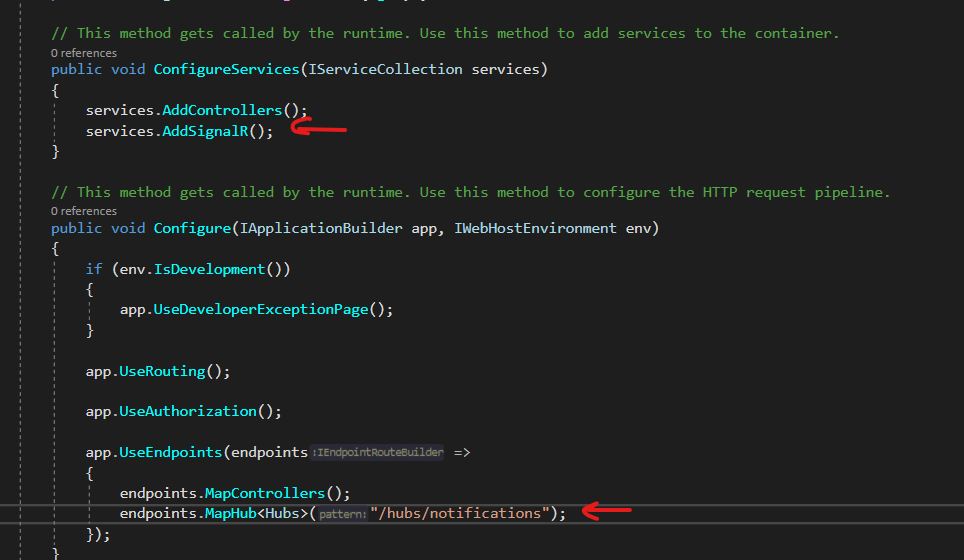
Code the Client UI
For front-end part I will using a simple React app with a TypeScript. I am using a create-react-app boilerplate for this:
npx create-react-app my-app --template typescript
We also need to add a package for SignalR from Microsoft:
yarn add @microsoft/signalr
The entire code should look something like this:
import { HubConnection, HubConnectionBuilder } from "@microsoft/signalr";
import { Button, Input, notification } from "antd";
import React, { useEffect, useState } from "react";
export const Notify = () => {
const [connection, setConnection] = useState<null | HubConnection>(null);
const [inputText, setInputText] = useState("");
useEffect(() => {
const connect = new HubConnectionBuilder()
.withUrl("http://localhost:51130/hubs/notifications")
.withAutomaticReconnect()
.build();
setConnection(connect);
}, []);
useEffect(() => {
if (connection) {
connection
.start()
.then(() => {
connection.on("ReceiveMessage", (message) => {
notification.open({
message: "New Notification",
description: message,
});
});
})
.catch((error) => console.log(error));
}
}, [connection]);
const sendMessage = async () => {
if (connection) await connection.send("SendMessage", inputText);
setInputText("");
};
return (
<>
<Input
value={inputText}
onChange={(input) => {
setInputText(input.target.value);
}}
/>
<Button onClick={sendMessage} type="primary">
Send
</Button>
</>
);
};